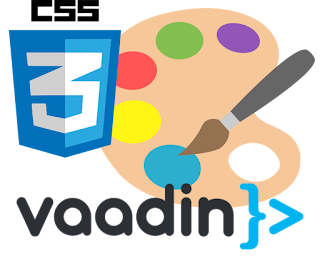
CSS Theming & Styling in Vaadin 14
Last week, the new LTS version of the presentation framework was announced.
While you can find more information about the new features in the official site, I would like to focus on one specific feature that I’ve found interesting: styles management.
Across the years we saw different approaches in how to handle styles in Vaadin applications.
In Vaadin 6 you could just modify styles using plain CSS. Of course you had two choices: either build everything from scratch, or inherit the built-in themes like Reindeer, Runo or Chameleon. That inheritance scheme was based in the @import css statement, which is the basis of the cascading part of the theming technology.
The problem with this approach was that the customization was difficult, because the complexity of the rendered DOM, and the lack of variables that could help in modifying certain aspects of the base themes such as sizing and spacing, that several rules had in common.
That was one of the reasons that lead to changing the underlying theming technology in favor of SASS (which stands for "Syntactically Awesome Style Sheets") themes in Vaadin 7.
SASS is an extension of CSS that enables you to use variables, nested rules, inline imports and more. It also helps to keep things organised and allows you to create style sheets faster. It works by adding a post-processing step, that will render the whole SASS hierarchy in a single plain css final file.
With this power up in the themes architecture, Valo was born. It was a brand new theme with important customization features. You were able to change specific aspects with the use of SASS variables and rules, so the theme personalizations were significantly smaller, simpler and clearer.
The downside was that making simple changes in the css, would mean to change scss files, and re-generate the final styles.css file on the fly, adding a small overhead to development cycle.
With the advent of Vaadin 10 and Web Components, everything changed. Now GWT was no longer responsible for rendering the client-side components, and the DOM structure was significantly simpler.
With the brand new, browser-supported, frontend technologies, the possibility of using new CSS 3 features was at hand. That meant that variables, clear inheritance and many other features could be used without a css post-processor engine.
Of course there are new big things to solve, like web component style encapsulation. Shadow DOM styling and more.
With this in mind, and the new Vaadin version based on these new technologies, the new theme Lumo appeared.
Lumo is an evolving design system foundation for modern web applications. It offers many customization paths, with the help of css variables.
Also later Material theme was released, with the same technical approach as Lumo.
Customizing them was just a matter of adding HTML files with a specific structure, that allowed the modification of not only global styles, but also styles that were to be injected into specific components’ Shadow DOM.
There were some caveats with this approach. As an example, the structure of these files was not so easy to remember, and existent tooling had to support this structure. Another problem was that you needed to import other style dependencies.
With the release of the new LTS version, a new feature was introduced: Simplified styling of application and components with @CssImport. This annotation removes the complexity of the previous version. Now you can easily import plain .css files directly into java code, without the need of post processing engines, while enjoying the new features of CSS3 & Web Components.
Some of the benefits of this approach:
While you can find more information about the new features in the official site, I would like to focus on one specific feature that I’ve found interesting: styles management.
Across the years we saw different approaches in how to handle styles in Vaadin applications.
In Vaadin 6 you could just modify styles using plain CSS. Of course you had two choices: either build everything from scratch, or inherit the built-in themes like Reindeer, Runo or Chameleon. That inheritance scheme was based in the @import css statement, which is the basis of the cascading part of the theming technology.
The problem with this approach was that the customization was difficult, because the complexity of the rendered DOM, and the lack of variables that could help in modifying certain aspects of the base themes such as sizing and spacing, that several rules had in common.
![]() |
Styling in Vaadin 6: Complex DOM Structure & lack of variables |
That was one of the reasons that lead to changing the underlying theming technology in favor of SASS (which stands for "Syntactically Awesome Style Sheets") themes in Vaadin 7.
SASS is an extension of CSS that enables you to use variables, nested rules, inline imports and more. It also helps to keep things organised and allows you to create style sheets faster. It works by adding a post-processing step, that will render the whole SASS hierarchy in a single plain css final file.
With this power up in the themes architecture, Valo was born. It was a brand new theme with important customization features. You were able to change specific aspects with the use of SASS variables and rules, so the theme personalizations were significantly smaller, simpler and clearer.
![]() |
Styling in Vaadin 7 & 8: SASS features |
With the advent of Vaadin 10 and Web Components, everything changed. Now GWT was no longer responsible for rendering the client-side components, and the DOM structure was significantly simpler.
With the brand new, browser-supported, frontend technologies, the possibility of using new CSS 3 features was at hand. That meant that variables, clear inheritance and many other features could be used without a css post-processor engine.
Of course there are new big things to solve, like web component style encapsulation. Shadow DOM styling and more.
With this in mind, and the new Vaadin version based on these new technologies, the new theme Lumo appeared.
Lumo is an evolving design system foundation for modern web applications. It offers many customization paths, with the help of css variables.
Also later Material theme was released, with the same technical approach as Lumo.
Customizing them was just a matter of adding HTML files with a specific structure, that allowed the modification of not only global styles, but also styles that were to be injected into specific components’ Shadow DOM.
![]() |
Styling in Vaadin 10+: Web Components Theming |
There were some caveats with this approach. As an example, the structure of these files was not so easy to remember, and existent tooling had to support this structure. Another problem was that you needed to import other style dependencies.
With the release of the new LTS version, a new feature was introduced: Simplified styling of application and components with @CssImport. This annotation removes the complexity of the previous version. Now you can easily import plain .css files directly into java code, without the need of post processing engines, while enjoying the new features of CSS3 & Web Components.
![]() |
Styling in Vaadin 14+: @CssImport elegance |
Some of the benefits of this approach:
- The file reference is simpler
- You can include multiple imports
- The “themeFor” is specified in the annotation
- No need to import dependencies in css
- No more <custom-style>, <dom-module> or <template> tags
Now we should expect better looking Vaadin applications with simplified styling!